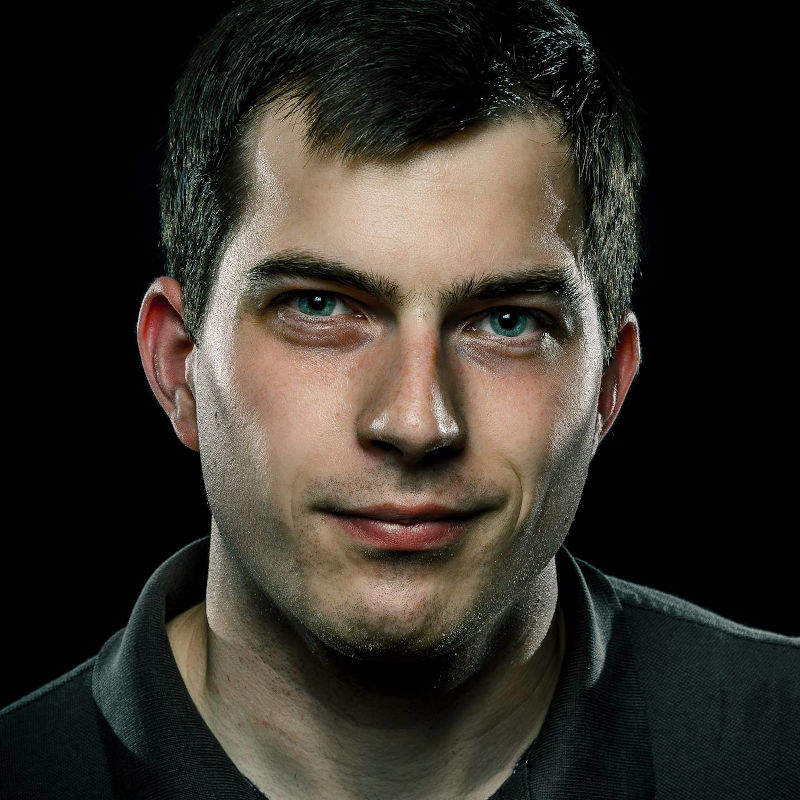
Johannes Wienke
software architect
I am a software architect and software engineer focusing on the architecture and accompanying software development processes for complex distributed systems such as microservice architectures. Besides my professional work, I actively contribute to the open source community.